Databases for Android Applications: A Comprehensive Overview
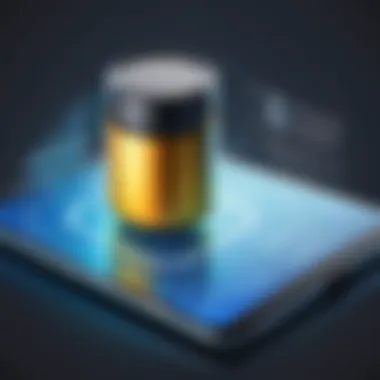
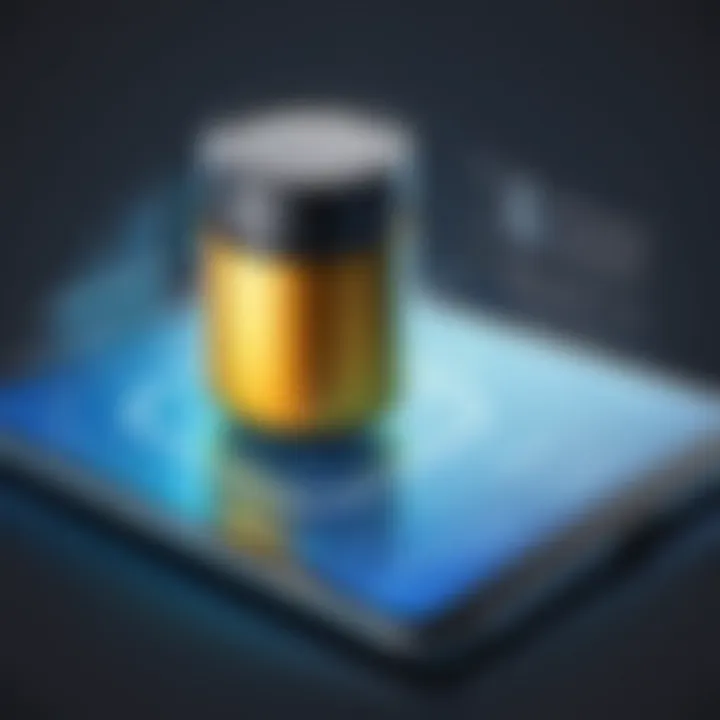
Intro
As the demand for Android applications continues to grow, understanding the various database options becomes essential for developers. Android apps often require efficient data storage and retrieval to ensure smooth performance and user experience. In this landscape, several database solutions exist, each with unique characteristics. This overview aims to dissect options such as SQLite, Room, and cloud solutions.
Developers must consider various factors when selecting a database, including ease of integration, flexibility, and performance optimization. This guide will also highlight best practices for database management to maintain optimal functionality in Android applications.
Preface to Databases in Android Development
Databases play a crucial role in the landscape of Android development. They allow mobile applications to manage and store data efficiently. This functionality is not merely a feature but a necessity for modern apps that require data persistence and retrieval. A well-designed database can enhance user experience by providing quick access to information and supporting complex functionalities.
An essential aspect of Android databases is how they accommodate the diverse needs of applications. Developers must choose suitable database technologies according to their project requirements. Different applications have varying data management needs, ranging from simple to complex. Mobile applications, such as games, e-commerce, and social media, rely on databases to handle user data, transaction records, and dynamic content.
One significant benefit of utilizing databases in Android development is improved performance. When an application accesses data from a database, it can retrieve and manipulate information more rapidly than accessing information from other sources like hard-coded values or files. This efficiency is particularly important for applications that need to operate smoothly and responsively.
There are also various considerations that developers must keep in mind. Factors such as data integrity, security, and scalability are vital. For instance, ensuring that data remains consistent and accurate is a top priority. Moreover, as user's data grows over time, developers must ensure that the chosen database can scale appropriately without compromising performance.
In summary, the introduction of databases in Android development is integral to building effective, user-friendly applications. Understanding the types of databases available and their applications in real-world scenarios is essential for developers. This section lays the foundation for further exploration of specific database technologies that can enhance Android development.
Importance of Databases for Mobile Applications
Databases play a crucial role in the functionality of mobile applications. They are essential for managing, storing, and retrieving data efficiently. In Android development, selecting the right database can impact the app's performance, reliability, and user experience.
Mobile applications rely heavily on data. Users expect seamless access to their information, whether it is social media, photos, or transactions. A well-implemented database ensures that this data is managed efficiently. Developers must consider the various database options available, such as SQLite, Room, or cloud-based solutions, each providing distinct advantages and limitations.
Leveraging databases allows for complex data structures and queries. Applications can handle relationships between data entities, ensuring data integrity and consistency. This capability is vital for any app handling user profiles, preferences, or interactions. A database encourages organized data management, aiding in both development and maintenance phases.
Benefits of Using Databases in Mobile Apps:
- Data Persistence: Databases provide long-term data storage, ensuring that user information is retained across sessions.
- Scalability: As the application grows and attracts more users, databases can handle increased loads, making scaling easier.
- Complex Queries: With structured databases, developers can perform complex queries swiftly, improving app performance.
- Data Security: Safe storage and management of sensitive information are crucial in today’s data-conscious environment. A robust database approach includes built-in security measures.
Mobile applications often deal with dynamic data, which necessitates the need for a database. Whether users are creating, updating, or deleting data, the database must manage these actions efficiently. An app that integrates well with its database can provide faster responses and is less prone to data corruption, thus enhancing user satisfaction.
A properly designed database architecture is as important as the application logic when it comes to mobile apps.
Considerations When Choosing a Database:
- Data Type: The nature of data being handled can influence the choice of database. For instance, relational data might be better suited for SQLite.
- Operational Complexity: If the app requires complex transactions, a full SQL database may be advantageous.
- Performance Needs: For performance-sensitive applications, evaluating the speed of data access and throughput is critical.
Overview of Database Management Systems for Android
In the realm of Android development, the choice of a database management system (DBMS) plays a crucial role in the overall performance and functionality of mobile applications. This section delves into the key components and benefits of various database systems specifically designed for Android. By understanding these systems, developers can make informed choices that align with their project requirements.
A database management system provides the necessary tools to create, retrieve, update, and delete data. For Android applications, these functionalities enable smooth user interactions and ensure data is handled efficiently. Typically, there are three main types of database management systems in Android: relational databases, NoSQL databases, and cloud-based solutions. Each type has distinct characteristics, offering various advantages and challenges that need evaluation based on application needs.
1. Relational Databases
These are structured systems that use tables to organize data. SQLite is the most popular relational database for Android applications. It is lightweight and integrated directly into the Android system, facilitating portability and ease of use.
- Key Benefits:
- Considerations:
- Data integrity is enhanced through enforced relationships between data tables.
- Structured Query Language (SQL) offers powerful querying capabilities.
- Complexity arises when dealing with large datasets or intricate relationships.
2. NoSQL Databases
NoSQL databases, such as Firebase Realtime Database, cater to unstructured data and focus on scalability. They offer flexibility in data storage, making it easier to accommodate changing app requirements.
- Key Benefits:
- Considerations:
- They provide high availability and fault tolerance.
- JSON data format promotes straightforward integration with web-based solutions.
- NoSQL databases might lack some features around transaction consistency found in relational systems.
3. Cloud-Based Solutions
Cloud databases have gained traction due to their on-demand access to data from anywhere, enhancing collaboration among development teams. Providers like Google Cloud Firestore allow for real-time updates and offline data management.
- Key Benefits:
- Considerations:
- Automatic scaling to manage fluctuating workloads.
- Reduced operational costs by eliminating the need for maintaining local database servers.
- Dependency on internet connectivity can affect performance, especially in remote areas.
In summary, selecting the right database management system for Android applications requires consideration of various factors, including data structure, scalability, and specific project needs. A thorough understanding of these categories enables developers to implement efficient solutions that enhance user experiences.
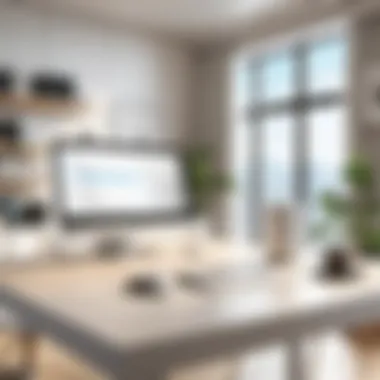
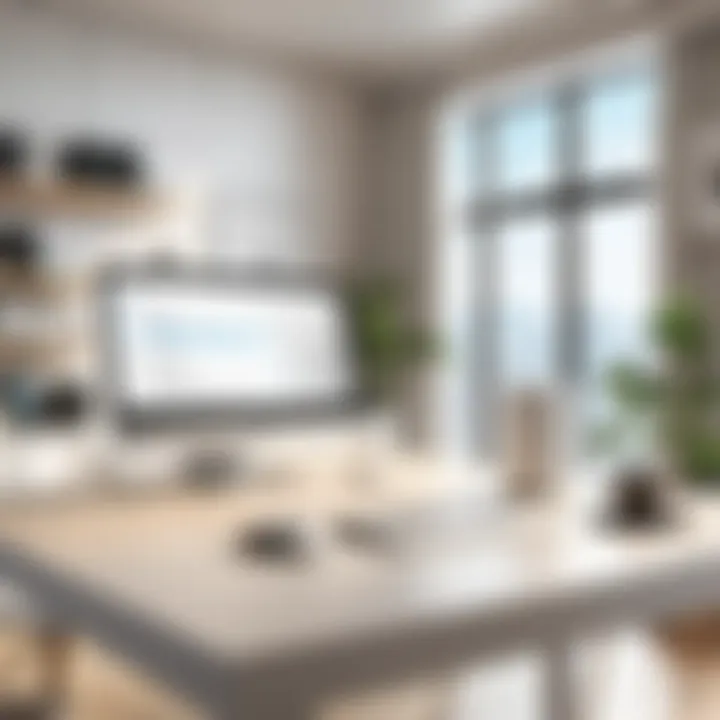
This overview serves as a foundation for further exploration into specific database options such as SQLite and Room, highlighting their unique features and advantages. Each system offers potential that can significantly impact the development process and final product. A thoughtful choice in DBMS may very well determine the application's success.
SQLite: The Built-In Database for Android
SQLite serves as the default database management system for Android applications. Its integration into the Android ecosystem is critical, as it provides a lightweight and efficient means for local data storage without needing internet access. SQLite is structured as a relational database and operates without the support of a separate server process. This autonomy ensures that it can be easily embedded within applications, making data management straightforward and efficient for developers.
Key Features of SQLite
- Self-Contained: SQLite is a self-contained database, meaning that it requires minimal setup and administration. Developers can bundle the SQLite library with their applications seamlessly.
- Cross-Platform Compatibility: SQLite is compatible with various operating systems, enhancing the portability of Android applications across different devices.
- Lightweight: The entire database engine is less than a megabyte in size, making it an excellent option for mobile apps that have limited storage capacity.
- Full-Text Search: SQLite supports full-text search (FTS) capabilities, allowing developers to implement advanced search features within their apps.
- ACID Compliance: SQLite is ACID-compliant (Atomicity, Consistency, Isolation, Durability). This means transactions are processed reliably, preserving data integrity throughout operations.
Advantages of Using SQLite
- Performance: SQLite operates remarkably fast for read and write operations due to its efficient query processing and indexing mechanisms.
- Concurrency: Its file-based architecture allows for concurrent access, meaning multiple threads can access the database without significant performance degradation.
- Zero Configuration: There is no need for complex setup. Developers can access and manage databases without extensive database administrator (DBA) involvement.
- Data Locality: As a local storage solution, SQLite enables applications to function offline, essential for user experience in mobile environments where connectivity can be inconsistent.
Limitations of SQLite
- Scalability Issues: While SQLite performs well with small to medium-sized datasets, its performance can degrade with large datasets. For applications with extensive data requirements, alternative solutions may be necessary.
- Limited Concurrent Write Access: SQLite allows multiple threads to read the database concurrently but only permits a single write operation at a time. This can create challenges in highly concurrent applications.
- No Built-in User Management: Unlike traditional database management systems, SQLite does not have built-in user management features. Developers must implement their security measures to manage data access and control.
"SQLite is an excellent option for developers looking for ease of use and efficiency in managing application data. However, it is essential to assess its limitations in larger deployments."
Room: An Abstraction Layer over SQLite
Room provides an essential abstraction layer over SQLite, streamlining data management in Android applications. It serves to simplify database operations while maintaining the robust foundation of SQLite. As Android developers increasingly require more sophisticated data-handling capabilities, Room has emerged as a preferred choice. It allows for better organization of data access code and fosters cleaner architecture, making it particularly valuable in complex applications.
Architecture of Room
The architecture of Room consists of three key components: the Database, Entities, and Data Access Objects (DAOs).
- Database: This is the main component that holds the database and provides the connection between the database and the code. Developers can define the database with annotations to specify the entities it holds.
- Entities: These are the tables in your database, represented as classes. Each entity class corresponds to a table, with properties representing the columns.
- Data Access Objects (DAOs): DAOs serve as the main connection to the database. They provide methods for querying, inserting, updating, and deleting data. This separation allows developers to manage database interactions more effectively.
With this architecture, Room enables compile-time checks of SQL queries, ensuring potential errors are caught early, thereby reducing runtime exceptions.
Benefits of Room Framework
The Room framework offers several significant benefits for Android developers:
- Compile-time verification: Unlike raw SQL strings in SQLite, Room validates queries at compile-time. This feature reduces the potential for runtime errors.
- Easier migration processes: Room provides straightforward migration helpers, minimizing the complexities involved in updating database schemas.
- Reactive programming support: Room is integrated with LiveData and RxJava, allowing developers to observe and react to changes in the data without writing cumbersome boilerplate code.
- Increased code maintainability: By following an architecture that separates concerns, Room enhances the maintainability of the codebase. This allows for easier testing and refactoring in the long term.
Limitations and Considerations When Using Room
While Room provides many advantages, there are also limitations and considerations:
- Overhead: The abstraction layer adds some overhead compared to using SQLite directly. For very performance-critical applications, this could be a consideration.
- Complexity for simple use cases: For simple, straightforward applications, the additional structure and overhead of Room may not be necessary, possibly complicating implementations unnecessarily.
- Limited support for certain SQL features: Some advanced SQLite features may not be fully supported by Room, necessitating workarounds or direct SQLite features that could complicate the code base.
Choosing External Cloud-Based Databases
In the current landscape of mobile application development, the choice of database is crucial. As Android applications evolve, many developers turn to external cloud-based databases. This shift is driven by the need for greater scalability, flexibility, and easier management of data. Using cloud-based solutions allows developers to leverage remote servers to store and access data efficiently, promoting smoother application performance and user experiences.
Popular Cloud Database Solutions
Several cloud database solutions have gained popularity in the Android ecosystem. Among these, Firebase Realtime Database stands out for its real-time data syncing capabilities. This allows developers to facilitate real-time updates in applications, a crucial feature for chat apps and collaborative tools. Another notable option is Amazon DynamoDB, which is renowned for its high performance and scalability. Google Cloud Firestore also deserves mention for its rich querying capabilities and strong integration with Google's ecosystem. These solutions provide reliable services that adapt well to diverse application requirements.
- Firebase Realtime Database
- Amazon DynamoDB
- Google Cloud Firestore
Benefits of Cloud-Based Databases for Mobile Apps
Cloud-based databases offer numerous advantages for mobile applications. One major benefit is the ability to scale automatically. As user demand grows, cloud databases can adjust resources without major downtime. This is particularly essential for companies that anticipate rapid growth or seasonal fluctuations in usage.
Another significant advantage is reduced infrastructure costs. By utilizing cloud services, developers can minimize expenses associated with hardware and maintenance. In addition, cloud databases usually come with built-in security features. Data encryption and automated backups are often standard, enhancing user trust in data protection.
"Cloud-based databases are essential for modern applications, providing both flexibility and robustness that aligns well with today’s user expectations."
Drawbacks and Challenges of Cloud Databases
Despite the benefits, there are some drawbacks to consider. One of the primary concerns is latency. Remote data access can lead to delays, especially in areas with poor connectivity. This might not be ideal for applications requiring immediate responses.
Security is another critical consideration. While many cloud providers implement substantial security measures, developers must still ensure data privacy and adhere to regulations such as GDPR.
Cost is also an aspect that can escalate rapidly. While cloud solutions may seem less expensive initially, costs can increase with enhanced data storage and retrieval needs.
Integrating Databases into Android Applications
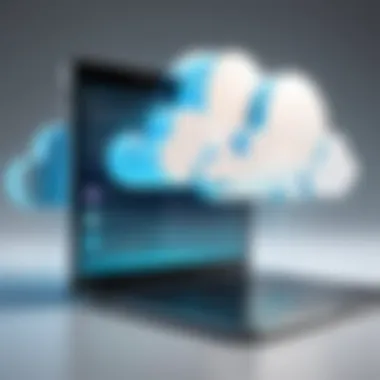
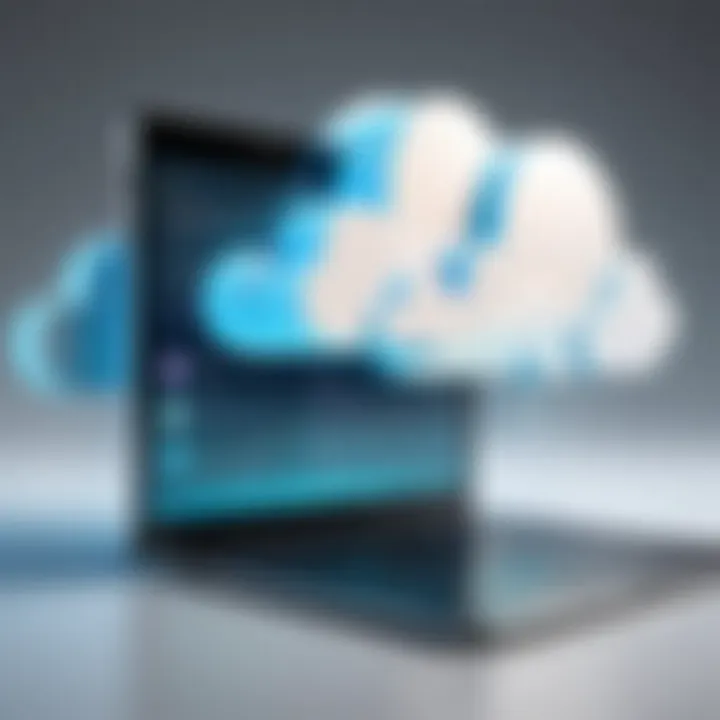
The integration of databases into Android applications is crucial for ensuring robust data management and performance. Mobile applications today often require efficient ways to store and retrieve data, which necessitates a solid understanding of how to seamlessly incorporate databases. A well-integrated database can enhance user experience, improve data reliability, and support the overall functionality of the application.
When developing an Android app, the choice of database and its integration affects various aspects—performance, data handling, and application scalability. Understanding these elements can significantly contribute to the success of a project, especially in a field where user expectations are at an all-time high.
Setup and Configuration Process
The first step in integrating a database into an Android application is the setup and configuration process. This involves multiple stages:
- Selecting the Right Database: Depending on the application requirements, developers can choose from SQLite, Room, or cloud-based solutions. Each option has its strength and weaknesses suitable for different scenarios.
- Including Necessary Dependencies: For SQLite or Room, developers need to add library dependencies to the Gradle file. This ensures the application has access to essential database functionalities. For example, Room requires:
- Creating Database Classes: Developers must define the database entities, including the data structure and the relationships among them. Annotations are often used to map these classes to database tables.
- Configuring Database Access: Developers should set up database access methods, ensuring proper insertion, deletion, and updating of data. This often involves implementing a DAO (Data Access Object).
- Handling Database Versioning: As applications evolve, database schemas may require updates. Managing these changes through version control is essential. This involves incrementing the database version and defining migration strategies to ensure data integrity.
Setting up a database correctly can provide a strong foundation for the application.
Data Access and Management Techniques
After setup, focus shifts toward data access and management techniques. Efficient data management is key to optimizing application performance. Consider the following aspects:
- DAO Interfaces: DAOs encapsulate the data access logic and provide an abstraction layer to interact with the database. This promotes cleaner code and easier maintenance.
- Async Operations: To ensure user interfaces remain responsive, database operations should be executed asynchronously. Utilizing LiveData or Kotlin Coroutines can help in managing background operations effectively.
- Data Observability: Implementing observable data patterns helps in keeping the UI updated with changes in the database. Using LiveData in Room is an effective way to achieve this.
- Transactions: When performing a series of database operations, wrapping them in a transaction ensures that they are executed atomically. This prevents partial updates and helps maintain data integrity.
- Data Querying: Crafting efficient queries is essential. With Room, developers can use annotations like @Query to create complex SQL queries in a more readable format.
By understanding these techniques, developers can ensure that their applications are not only functional but performant. Proper integration of databases will lead to smoother user experiences and more effective data management.
Data Security and Privacy Considerations
The role of data security and privacy in Android applications is critical. As mobile devices become the primary means for accessing the internet, they store invaluable user data. This data can range from personal details to financial information. Therefore, implementing robust security measures is not just a choice but a necessity for developers. Without proper security protocols, applications are vulnerable to data breaches and unauthorized access, leading to a loss of user trust and potential legal ramifications.
Data security ensures that sensitive information is protected from unwarranted access or alterations. Privacy considerations, on the other hand, focus on how user data is collected, processed, and stored. Developers must be aware of both elements to build applications that are not only functional but also dependable.
Best Practices for Data Protection
To enhance data security in Android applications, developers should adhere to several best practices:
- Implement Encryption: Encrypting data both in transit and at rest helps protect sensitive information. Utilizing libraries such as SQLCipher can provide additional security for SQLite databases.
- Use Secure Coding Techniques: Following guidelines such as the OWASP Mobile Security Testing Guide can minimize vulnerabilities in application code.
- Limit Data Access: Employ the principle of least privilege. Only request permissions necessary for the app’s functionality.
- Regular Security Audits: Periodic assessments of the application and its dependencies can identify and rectify potential security flaws.
- Keep User Updated: Regular updates not only improve functionality but also patch security vulnerabilities. Ensure the app can receive timely updates from Google Play.
"A chain is only as strong as its weakest link." This adage holds particularly true in data security.
Regulatory Compliance for Mobile Apps
Regulatory compliance is another critical aspect that demands attention. Various laws govern data protection and privacy, such as the General Data Protection Regulation (GDPR) in Europe and the California Consumer Privacy Act (CCPA) in the United States. These regulations outline specific requirements regarding how user data should be handled.
- User Consent: Obtain explicit consent from users before collecting their personal data.
- Transparency: Clearly inform users about how their data will be used, processed, and stored.
- Right to Access and Deletion: Users should be able to request access to their data and have the option to delete it if desired.
- Data Breach Notification: In case of a data breach, inform affected users promptly and take necessary action to mitigate risks.
The implications of failing to comply with such regulations can be severe, including heavy fines and reputational damage. Therefore, staying informed about legal landscapes is necessary for any developer seeking to create secure and compliant Android applications.
Performance Optimization for Android Databases
In the realm of Android application development, performance optimization for databases plays a crucial role. How an app manages data significantly influences its responsiveness, resource utilization, and user satisfaction. For developers, ensuring efficient database performance means implementing strategies that enhance speed, reduce latency, and optimize resource consumption. This process is not merely about code; it also revolves around infrastructure decisions and user experiences.
When a database operates optimally, it contributes to the overall smoothness of the application. A well-optimized database can handle increased loads, process transactions faster, and return queries in nanoseconds rather than seconds. This efficiency is vital for business applications where time is often equated with revenue. Specific considerations, such as reducing unnecessary database calls and managing data effectively, are paramount in achieving the desired performance levels.
Techniques for Enhancing Performance
To enhance performance in Android databases, developers can employ several effective techniques:
- Indexing: Creating indexes on frequently queried columns can significantly speed up data retrieval processes. Indexes act as shortcuts, allowing the database engine to locate data without scanning entire tables.
- Using Batching: Instead of executing multiple queries separately, batching allows developers to send several statements in a single request. This reduces the overhead associated with network communication and can speed up operations.
- Caching Results: By storing frequently accessed data in memory, developers can minimize database calls. This greatly boosts performance, especially for read-heavy applications.
- Optimizing Queries: Writing efficient SQL queries is essential. Avoiding complex joins and unnecessary columns can lead to faster response times.
- Database Transactions: Utilizing transactions properly ensures that multiple operations are completed successfully, enhancing reliability while improving performance through reduced locking overhead.
"Optimizing database performance not only improves application speed but also enhances user experience."
Common Pitfalls and How to Avoid Them
Despite the best intentions, developers often encounter common pitfalls when optimizing database performance. Recognizing these issues early can lead to better decision-making:
- Over-Indexing: While indexing helps with speed, having too many indexes can actually slow down write operations. Developers must find a balance in their indexing strategy.
- Neglecting Cleanup: Old and unnecessary data can bloat a database, worsening performance. Regularly reviewing and purging data can prevent this from happening.
- Ignoring SQL Query Plans: Not analyzing the execution plan of SQL queries can lead to performance problems that are hard to detect. Examining the plan can provide insight into how to optimize queries effectively.
- Failing to Use Prepared Statements: Using raw SQL statements can open the door to SQL injection attacks and lead to suboptimal performance. Prepared statements are a safer and often more efficient alternative.
- Underestimating Connection Management: Not properly managing database connections can lead to resource leaks and performance bottlenecks. It's crucial to close connections when they are no longer needed, and consider connection pooling for scalability.
By understanding and addressing these common challenges, developers can successfully optimize performance while utilizing Android databases. This not only enhances application quality but also leads to increased user satisfaction.
Case Studies: Successful Database Implementations
Case studies examining successful database implementations in Android applications serve a dual purpose. First, they provide concrete examples of how various database systems behave in real-world scenarios. Second, they highlight the advantages and challenges developers might face when using such systems. Ultimately, these case studies offer lessons that guide future decisions in database selection and implementation.
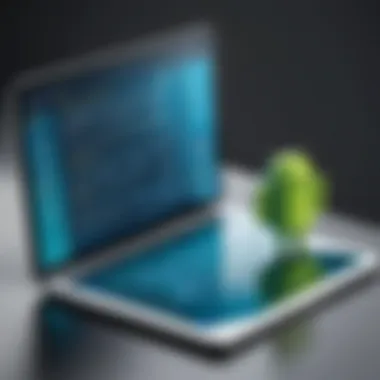
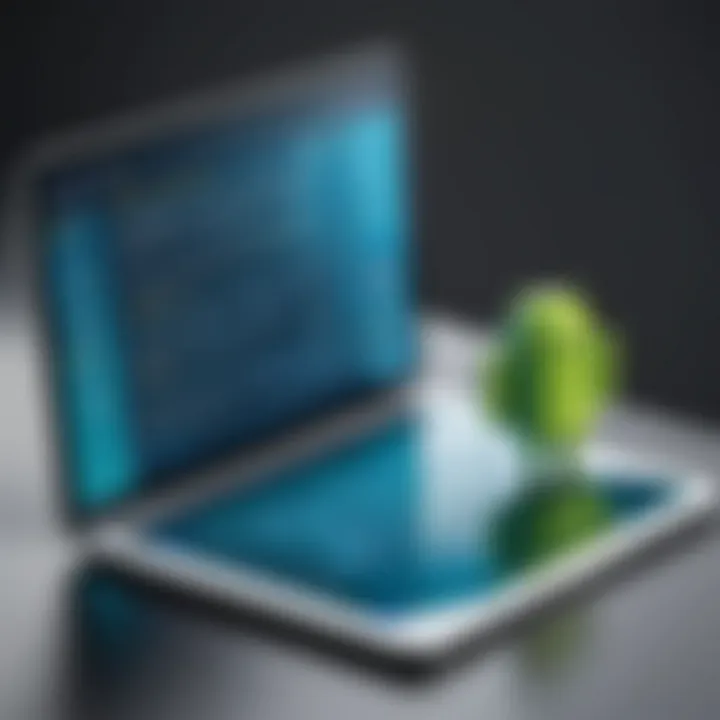
Analysis of Mobile Apps Utilizing SQLite
SQLite is widely used in many mobile applications due to its simplicity and lightweight nature. For instance, applications like WhatsApp and Instagram leverage SQLite to manage local data storage efficiently. These applications benefit from SQLite's fast read and write operations, allowing for seamless user experiences.
Key Factors to Consider:
- Local Data Management: SQLite allows apps to function offline and sync data later, which enhances user convenience.
- Compact Size: As a self-contained database, SQLite does not require server processes, making it easy to include within the app binary.
The integration process involves creating a database schema and implementing CRUD operations. Developers often use SQLiteOpenHelper to manage database creation and version management.
Examination of Applications Using Room
Room brings a higher level of abstraction to SQLite, which results in a more streamlined development process. Many popular applications, such as Evernote, utilize Room to achieve better performance and maintainability. Room facilitates direct interaction with SQLite through annotations, enhancing code readability.
Essential Aspects:
- Better Compile-time Verification: Room checks SQL queries at compile time, reducing runtime errors.
- LiveData Support: It integrates well with Android Architecture Components, allowing automatic UI updates as data changes.
The design allows developers to focus more on writing clean and organized code while taking advantage of Room's enhanced functionalities.
Insights from Cloud-Based Database Implementations
Cloud-based databases, such as Firebase Realtime Database and Amazon DynamoDB, offer unique advantages for Android applications. These systems are highly scalable and support real-time data syncing across devices. Applications like Uber and Netflix utilize cloud databases to support their dynamic data needs despite high volumes of user engagement.
Significant Points:
- Scalability: Cloud databases can easily accommodate growing data without significant reconfiguration.
- Performance: They often provide better performance in scenarios involving concurrent users.
Implementing a cloud database requires careful planning regarding data structure, security, and cost management to optimize resource utilization.
"Successful database implementation depends not only on technology but also on understanding project-specific data needs and user interactions."
Future Trends in Database Technologies for Android
The landscape of database technologies continues to evolve, particularly in the realm of Android development. Trends dictate the direction in which the industry moves, impacting the choices developers make and the innovations they implement. Understanding these trends is crucial not only to keep up with the competition, but also to ensure best practices in performance, scalability, and user experience in mobile applications.
Emerging Technologies and Innovations
Several emerging technologies are shaping the future of database solutions for Android. Some noteworthy elements include:
- NoSQL Databases: Systems like Firebase Realtime Database and MongoDB are gaining popularity. They offer flexibility and can handle unstructured data effectively, which is increasingly relevant in mobile applications.
- Graph Databases: These databases, such as Neo4j, enable complex data relationships to be queried efficiently. They become essential for applications that require intricate connections between data, such as social networking apps.
- Blockchain Integration: With the rise of cryptocurrency and decentralized applications (dApps), blockchain technology is finding ways into mobile databases. It provides enhanced security and transparency, which appeal to users demanding higher data integrity.
Implementing these technologies can provide advantages such as scalability, faster query responses, and better alignment with modern development practices.
Potential Impacts on Android Development
The aforementioned trends have significant implications for Android development. Development teams need to consider how these technologies will influence their projects:
- Development Agility: The flexibility of NoSQL databases allows developers to iterate faster through testing and product cycles. This can help teams respond to user feedback more quickly.
- User Experience: The integration of advanced technologies may lead to better app performance, which directly enhances overall user satisfaction. When databases speed up data retrieval and processing, users benefit from smoother interactions.
- Security Considerations: As databases evolve, the focus on security becomes even more critical. Developers need to adapt their methods to implement security features inherent in new technologies while also adhering to regulatory requirements.
In summary, keeping abreast of existing and emerging database technologies is vital. By integrating trends such as NoSQL and blockchain, developers can create superior Android applications that meet the needs of modern users while preparing for a future where adaptability and innovation are essential for success.
Understanding and anticipating these trends allows developers to stay ahead while ensuring their applications remain relevant and efficient.
Thus, it is essential for tech-savvy professionals in the field to continually educate themselves and explore these advancements to harness the potential they bring to Android application development.
Epilogue and Recommendations
In summary, selecting the appropriate database for an Android application is a crucial decision that can greatly influence the overall functionality and performance of the app. This article has explored different database options including SQLite, Room, and various cloud-based solutions. Each option comes with its unique attributes and challenges, making it essential to consider the specific requirements of your project before making a choice.
Choosing the Right Database for Your Project
To decide on the right database, consider the following factors:
- Data Complexity: Evaluate how complex the data interactions will be. For simple, local storage needs, SQLite may suffice. If you need a more structured environment, Room could provide the necessary abstraction.
- Scalability: If you anticipate rapid growth or massive data handling, consider a cloud-based database like Firebase or Amazon DynamoDB, which can easily scale as your needs evolve.
- Offline Capability: Think about whether your application needs to function offline. SQLite offers a local storage solution, while some cloud databases might not support offline use as effectively.
- Integration Ease: Analyze the ease of integration with existing systems or technologies. Room, for instance, seamlessly integrates with other Android lifecycle components.
Making an informed choice here is essential for app performance and user experience.
Final Thoughts on Database Implementation
Implementing the chosen database effectively requires attention to detail. Keep in mind best practices for database management, like normalizing data to reduce redundancy, applying security methods for sensitive data, and regularly updating the database structure as the application evolves.
Also, maintain compliance with various data protection regulations, such as GDPR, which can impact how you handle user information.
"A well-chosen database can enhance not only the performance but also the user experience of your application. Therefore, invest time in the selection and implementation of your database architecture."
By understanding the nuances of each type of database and how they fit into your project, you can leverage these tools to build efficient, reliable, and robust Android applications.